RCS message
This documentation provides an overview of the message and action types supported by our messaging API. Each type is presented with a description and a JSON example to facilitate integration and use.
This comprehensive description and the JSON examples provide a deep insight into the possibilities of the RCS API. By using these different message and action types, developers can create a rich and interactive user experience that improves communication between businesses and customers.
Structure of the message
In principle, each message can consist of exactly one content type (text
, carousel
, richcard
or file
) and optionally contain suggested responses.
Properties
- Name
suggestions
- Type
- array
- Optional
- Optional
- Description
A list of reply suggestions and action suggestions that is displayed as a list below the last agent message. Maximum of 11 suggestions.
- Name
text
- Type
- string Union
- Description
A simple text message. Only makes sense here in connection with suggested answers, as otherwise you can simply send the text directly to our API as a simple string.
- objectUnion
carousel
A carousel is a collection of several richcards.- Name
width
- Type
- enum
- Description
Size of the carousel. Can be either
SMALL
orMEDIUM
.
- Name
richcards
- Type
- enum
- Description
A list of richcards.
- Name
richcard
- Type
- object Union
- Description
A richcard is a message enhanced with rich content. It can contain text, videos, a location and much more. Buttons are also possible with which the recipient can interact directly, for example to confirm a reservation or make a purchase.
- Name
file
- Type
- object Union
- Description
Send a file, such as an image, an audio file or a document, to the recipient.
{
"suggestions": [
// ...
],
// Only the following properties can be specified
// Either...
"text": "Your code is 1234",
// ...or...
"carousel": {
"width": "SMALL",
"richcards": [
{
// richcard object...
},
// ...
]
},
// ...or...
"richcard": {
"orientation": "horizontal",
"thumbnailImageAlignment": "right",
// ...
},
// ...or...
"file": {
"fileUrl": "https://acme.inc/images/image.png",
"thumbnailUrl": "https://acme.inc/images/thumbnail.png",
"forceRefresh": false
}
}
Richcard
- Name
title
- Type
- string
- Description
The title of the richcard
- Name
description
- Type
- string
- Description
The description of the richcard
- Name
orientation
- Type
- enum
- Optional
- Optional
- Description
This property is only to be specified when sending individual richcards. Can contain either
horizontal
orvertical
.
- Name
thumbnailImageAlignment
- Type
- enum
- Optional
- Optional
- Description
This property is only to be specified when sending individual richcards in horizontal layout. Can contain either
left
orright
.
- Name
file
- Type
- object
- Optional
- Optional
- Description
A file object.
- Name
suggestions
- Type
- array
- Optional
- Optional
- Description
A list of suggested answers and suggested actions that is displayed in the respective card. Maximum of 4 suggestions.
{
"title": "Title of the richcard",
"description": "Description of the richcard",
"orientation": "horizontal",
"thumbnailImageAlignment": "left",
"file": {
// File object
// ...
},
"suggestions": [
// list of suggested answers
]
}
Send file
Our API automatically caches files and thumbnails. If you want to make our API reload the image, just change it slightly. You could add a query parameter like ?timestamp=12345
or a hashtag.
The allowed mimetypes are: image/jpeg
, image/jpg
, image/gif
, image/png
, video/h263
, video/m4v
, video/mp4
, video/mpeg
, video/mpeg4
, video/webm
If the file is not part of a richcard but is specified in the root element, the mimetype application/pdf
is also permitted.
Properties
- Name
height
- Type
- enum
- Optional
- Optional
- Description
Only to be specified when used in a Richcard in vertical layout. Can be either
short
,medium
ortall
.
- Name
fileUrl
- Type
- string Union
- Description
Publicly accessible URL of the file. The RBM platform determines the MIME type of the file from the HTTP header
Content-Type
when the file is retrieved. Recommended maximum file size of 100 MB.
- Name
fileContents
- Type
- string Union
- Description
Contents of the file base64-encoded
- Name
thumbnailUrl
- Type
- string
- Optional
- Optional Union
- Description
Only for image and video files. Publicly accessible URL of the preview image. Maximum size 100 kB.
- Name
thumbnailContents
- Type
- string
- Optional
- Optional Union
- Description
Only for image and video files. base64-encoded contents of the thumbnail file. Maximum size 100 kB.
If you do not specify a thumbnail, an empty placeholder thumbnail is displayed until the user's device downloads the file. Depending on the user's settings, the file may not be downloaded automatically, but the user must tap a download button.
{
"height": "medium",
"fileUrl": "https://example.com/bild.jpg",
"thumbnailUrl": "https://example.com/thumbnail.jpg"
}
Answers and actions
The suggestions are only displayed if the associated agent message is the most recent message within the conversation (including agent messages and user messages). The user can tap on a suggested reply to send the text reply back to the agent or on a suggested action to start their own action on the device.
Properties
- Name
type
- Type
- enum
- Description
The type of response or action suggested. Can have one of the following values:
reply
dial
viewLocation
createCalendarEvent
openUrl
shareLocation
- Name
text
- Type
- string
- Description
Display text of the button.
- Name
postbackData
- Type
- string
- Description
Data to be returned when an action is triggered.
- Name
phoneNumber
- Type
- string
- Optional
- Optional
- Description
Only for the
dial
type. The phone number to be called.
- objectOptional
location
Only forviewLocation
. Contains the data of the location.- Name
latitude
- Type
- string
- Description
latitude
- Name
longitude
- Type
- string
- Description
longitude
- Name
label
- Type
- string
- Description
label
- objectOptional
calendarEvent
Only forcreateCalendarEvent
. Contains the data of the event.- Name
startTime
- Type
- string
- Description
The start time of the appointment. Common formats such as
19.06.2024 17:23
or2024-06-19 17:23
are accepted.
- Name
endTime
- Type
- string
- Description
The end time of the appointment. Common formats such as
19.06.2024 17:23
or2024-06-19 17:23
are accepted.
- Name
title
- Type
- string
- Description
The title of the calendar event.
- Name
description
- Type
- string
- Description
The description of the calendar event.
- Name
url
- Type
- string
- Optional
- Optional
- Description
Only for the
openUrl
type. The URL to be opened.
- Name
webviewMode
- Type
- string
- Optional
- Optional
- Description
Only for the
openUrl
type. The webview mode to be used when opening the URL. Can be eithertall
(website takes up three quarters of the screen),half
(website takes up half of the screen) orfull
(website takes up the full screen).
The webviewMode
property is only supported by some devices and may not work on all devices. If not supported, the default behavior is to open the URL in the device's default browser.
[
// Suggested response
{
"type": "reply",
"text": "yes",
"postbackData": "clicked_yes"
},
// Suggested call
{
"type": "dial",
"text": "call",
"postbackData": "clicked_call_button",
"phoneNumber": "49176123456789"
},
// view location
{
"type": "viewLocation",
"text": "view location",
"postbackData": "clicked_view_location_button",
"location": {
"latitude": 54.3233,
"longitude": 10.1228,
"label": "Kiel"
}
},
// Create calendar event
{
"type": "createCalendarEvent",
"text": "create event",
"postbackData": "clicked_call_button",
"calendarEvent": {
"startTime": "",
"endTime": "",
"title": "An important appointment",
"description": "We look forward to your visit."
}
},
// Open URL
{
"type": "openUrl",
"text": "Open",
"postbackData": "clicked_url_button",
"url": "https://www.example.com"
},
// share location
{
"type": "shareLocation",
"text": "share location",
"postbackData": "clicked_share_location_button"
}
]
Examples
Text message with suggested answers
Sends a simple text message with three suggested responses to the recipient. Text messages are the most basic
message types that can be used for direct communication of information, questions or instructions. You will
receive the value of postbackData
of the selected response in the webhook.
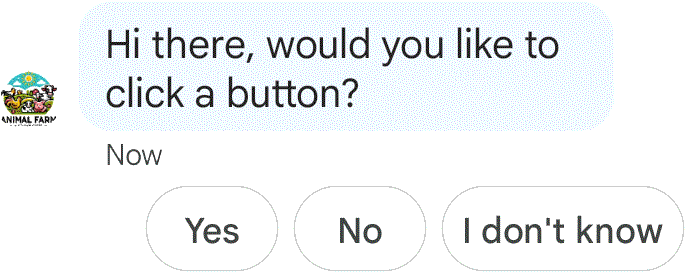
{
"text": "Hi there, would you like to click a button?",
"suggestions": [
{
"type": "reply",
"text": "Yes",
"postbackData": "clicked_yes"
},
{
"type": "reply",
"text": "No",
"postbackData": "clicked_no"
},
{
"type": "reply",
"text": "I don't know",
"postbackData": "clicked_dont_know"
}
]
}
Simple richcard with a picture
This example sends an image with the suggested action to display the image in the browser. Please note that the
suggestions
here are in the richcard instead of on the main layer. If they were on the main layer, the RCS
message would count as two messages and be more expensive.
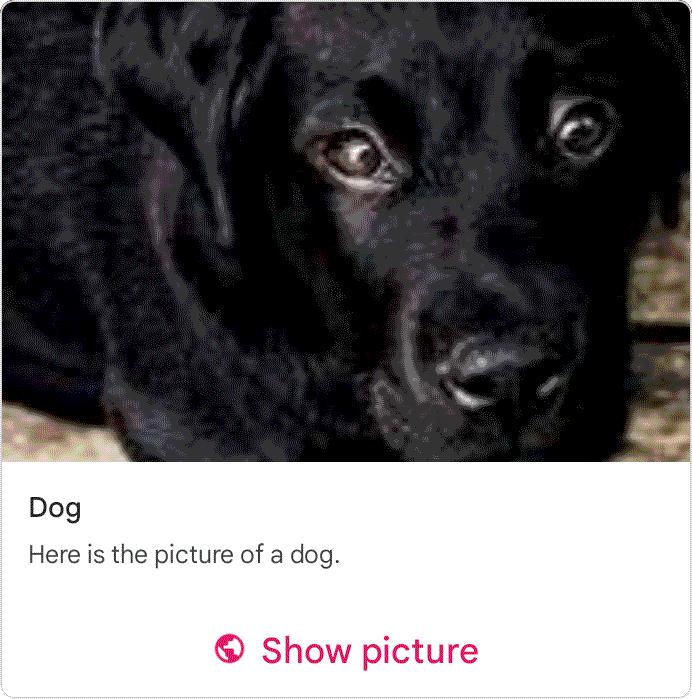
{
"richcard": {
"title": "Dog",
"description": "Here is the picture of a dog.",
"orientation": "vertical",
"file": {
"height": "tall",
"fileUrl": "https://picsum.photos/id/237/200/300",
"thumbnailUrl": "https://picsum.photos/id/237/50"
},
"suggestions": [
{
"type": "openUrl",
"text": "Show picture",
"postbackData": "clicked_url",
"url": "https://picsum.photos/id/237/800"
}
]
}
}
A vertical carousel
This example shows a carousel consisting of three individual, narrow cards in a horizontal layout, each with different heights.
Each of the three cards has a suggested action. For space reasons, the text of the suggested answer is not shown in full on the first card and the suggested action is not shown on the last card.
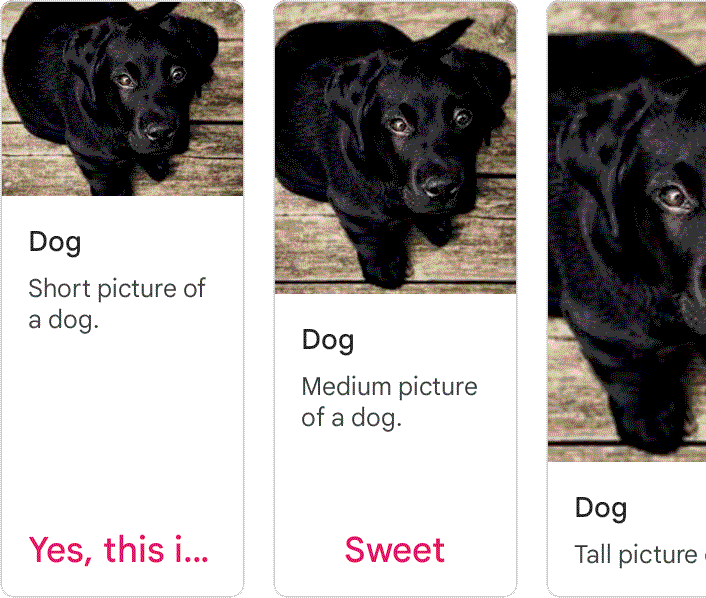
{
"carousel":
{
"width": "SMALL",
"richcards": [
{
"title": "Dog",
"description": "Short picture of a dog.",
"file":
{
"height": "short",
"fileUrl": "https://picsum.photos/id/237/300",
"thumbnailUrl": "https://picsum.photos/id/237/50"
},
"suggestions": [
{
"type": "reply",
"text": "Yes, this is a dog.",
"postbackData": "yes_this_is_a_dog_clicked"
}
]
},
{
"title": "Dog",
"description": "Medium picture of a dog.",
"file":
{
"height": "medium",
"fileUrl": "https://picsum.photos/id/237/300",
"thumbnailUrl": "https://picsum.photos/id/237/50"
},
"suggestions": [
{
"type": "reply",
"text": "sweet",
"postbackData": "sweet_dog_clicked"
}
]
},
{
"title": "Dog",
"description": "Tall picture of a dog.",
"file":
{
"height": "tall",
"fileUrl": "https://picsum.photos/id/237/300",
"thumbnailUrl": "https://picsum.photos/id/237/50"
},
"suggestions": [
{
"type": "reply",
"text": "yes",
"postbackData": "yes_clicked"
}
]
}
]
}
}